Background(배경)
background-image: url(절대,상대경로)
background-repeat: repeat | repeat-x | repeat-y 반복여부
background-position: 배경이미지 시작위치
background-size n% | contain | cover size작성시, position 을 꼭 작성해야한다!
contain: x/y축 어느 한 쪽이 꽉찰때까지만 확대/축소. 빈공간이 생길 수 있다.
cover: 영역을 모두 채울때까지 확대/축소. 이미지 짤릴 수 있다.
background-clip: border-box|padding-box|content-box: 배경이미지,컬러 영역
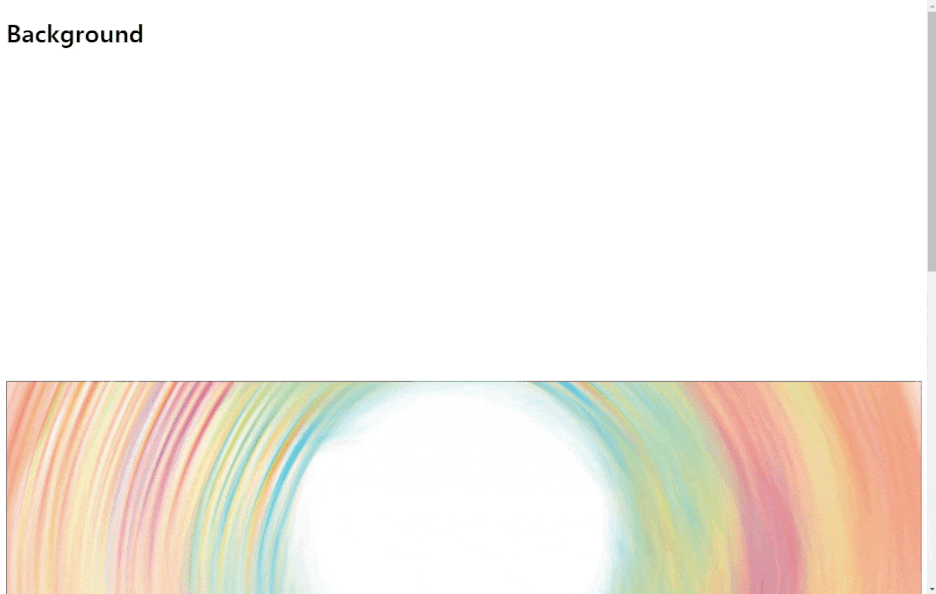
다음과 같은 스크롤시 사진은 고정되어있고 보이는 부분이 바뀌는 배경을 만들어본다.
<div id="only-bg"></div>
<style>
#only-bg {
width: 100%;
height: 800px;
border: 1px solid black;
background: coral url('../../../sample/image/bgsample.PNG') center center/cover no-repeat;
}
</style>
넓이를 100%로 했을때, 높이는 800px 이고, 1픽셀의 고정된 검정 실선이 주변에 표시된다.
background 설정을 보면, 배경의 색상 / 사진주소 / 배경이미지 정렬 / 가운데를기준으로 확장 / 반복없음 을 한번에 선언하였다.
cover를 이용하여 사진의 양쪽이 짤렸음을 알 수 있다.
position(위치설정)
문서상 배치를 결정하는 속성
- static(기본값)
- 문서에 정의된대로 배치하는 속성.offset(left,top,right,bottom) 무시
- float등을 이용해 좌우배치. static때는 안먹음
- relative
- 문서에 정의된대로 배치하는 속성. offset을 적용
- absolute
- 요소를 겹쳐서 표현이 가능. 가장 가까운 static이 아닌 부모 요소 기준offset을 적용
- fixed
- viewport기준으로 offset적용
- sticky(fixed 파생형)
- relative처럼 작동하다가 특정 offset(top:0px일때)다다르면 fixed처럼 작동
<h2 class="sticky">sticky</h2>
<h2>Static</h2>
<!--.outer>.first+.second+.third-->
<div class="outer">
<div class="first"></div>
<div class="second"></div>
<div class="third"></div>
</div>
<h2 class="sticky">relative</h2>
<div class="outer">
<div class="first"></div>
<div class="second relative"></div>
<div class="third relative"></div>
</div>
<h2 class="sticky">absolute</h2>
<div class="outer relative"><!--relative안쓰면 위에 지정됨. -->
<div class="first"></div>
<div class="second absolute"></div>
<div class="third absolute"></div><!--body기준 offset적용-->
</div>
<h2>fixed</h2>
<div class="fourth fixed"><!--화면에 고정됨 (기준이 view port)-->
</div>
.outer{
background-color: lightgray;
border: 1px solid #000;
margin-bottom: 200px;
}
.first{
width:300px;
height: 300px;
background-color: thistle;
}
.second{
width: 200px;
height: 200px;
background-color: violet;
left: 50px;
top: 50px;
}
.third{
width: 100px;
height: 100px;
background-color: magenta;
left: 100px;
top: 100px;
}
.fourth{
width: 100px;
height: 100px;
background-color: tomato;
right: 100px;
bottom: 100px;
border-radius: 50%;
}
.fixed{ position: fixed; }
.relative{ position: relative; }
.absolute{ position: absolute; }
.sticky{
position: sticky;
top:0px;
background-color: rosybrown;
z-index: 999;/*z축 기준 값이 클수록 위에 위치*/
}
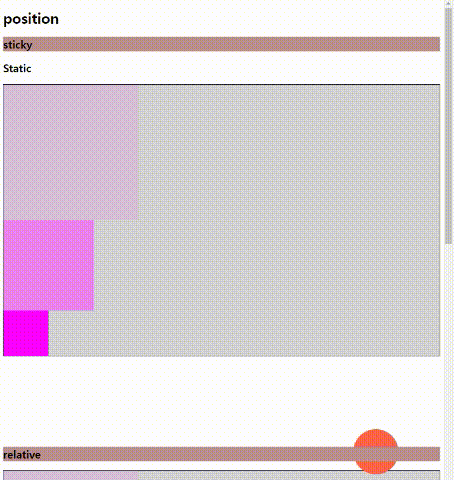
outer에 회색 배경색 화면이 적용된 것을 확인할 수 있다.
첫번째, 두번째, 세번째 색상을 선언했다. (점점 진해지게)
static의 경우 inline형식이 아니라 아래에 차곡차곡 쌓인다. (오프셋 적용안됨)
relative의 경우 left, top에 대한 offset이 적용된것이 드러나게 되는데, 바로 위 요소(가까운요소)와 관련되어 나오게 된다.
absolute는 제일 가까운 요소가 아닌 부모요소만 관련이 있다 (여기서는 background)
fixed의 경우 viewport를 기준으로 값을 지정하는데, fourth fixed(주황색 원)가 고정된 것을 확인할 수 있다.
마지막으로 위의 줄에 존재하는 sticky는 relative처럼 작동하다가, 그 다음 요소를 만나면 바뀌게 된다. 위의 경우 높이가 0px일때, 즉 가장 위에 존재할 때 바뀌도록설정되어 sticky와 relative가 바뀌는 것을 확인할 수 있다.
flexbox
- 부모요소 flex-container
- display : flex | inline-flex 기본배치 , 인라인배치
- flex-direction : row (기본값) | column 가로배치, 세로배치
- justify-content : flex-start | flex-end | space-between | space-evenly | space-around
- 메인축 정렬. flex-direction에서 방향이 결정되고, 정렬 방법이 정해진다.
- align-items : strech | baseline | center | flex-start | flex-end
- 크로스축 정렬. flex-direction의 방향과 교차축에 대해서 결정한다.
- strech는 flex-direction이 row(column)일 경우, height(weight) 속성이 없어야만 적용된다.
- flex-wrap : nowrap (기본값) | wrap 줄바꿈여부
- align-content : center | stretch | flex-start | flex-end | space-between | space-around
- 자식요소 flex-item
<!--ul.test>li{$}*10-->
<ul class="test">
<li>1</li>
<li>2</li>
<li>3</li>
<li>4</li>
<li>5</li>
<li>6</li>
<li>7</li>
<li>8</li>
<li>9</li>
<li>10</li>
</ul>
<style>
ul.test{
display: flex;
flex-direction: column;
justify-content: center;
align-items: center;
flex-wrap: wrap;
align-content: center;
list-style: none;
border:3px solid tomato;
height: 70vh;
padding: 0;
}
ul.test li{
width: 100px;
height: 100px;
background-color: orangered;
margin: 8px;
}
</style>
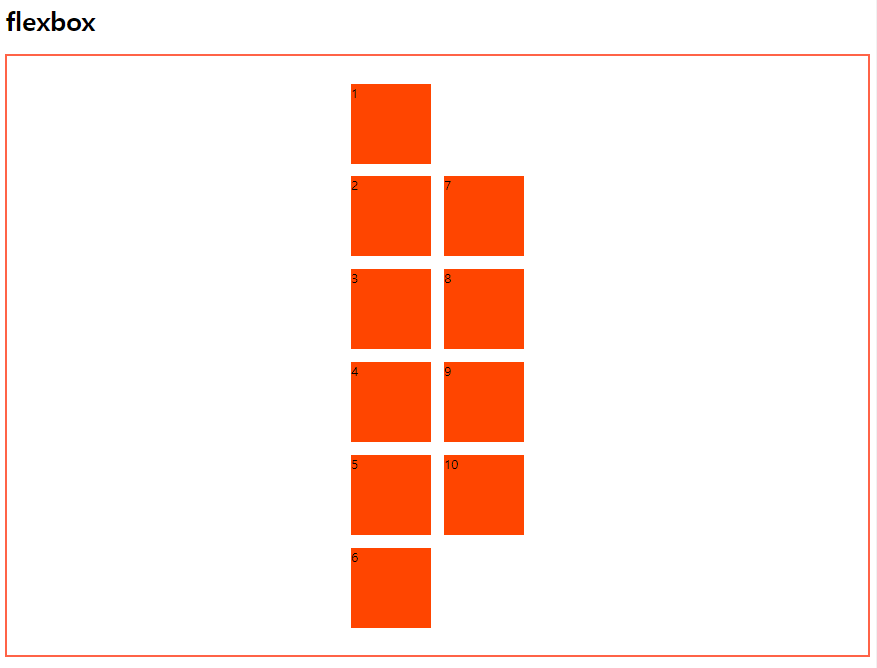
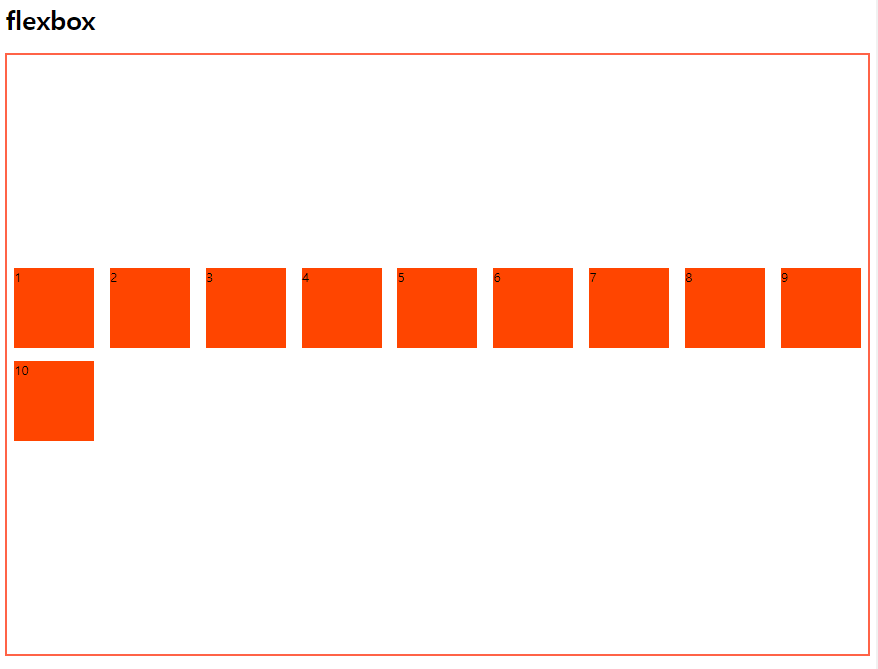
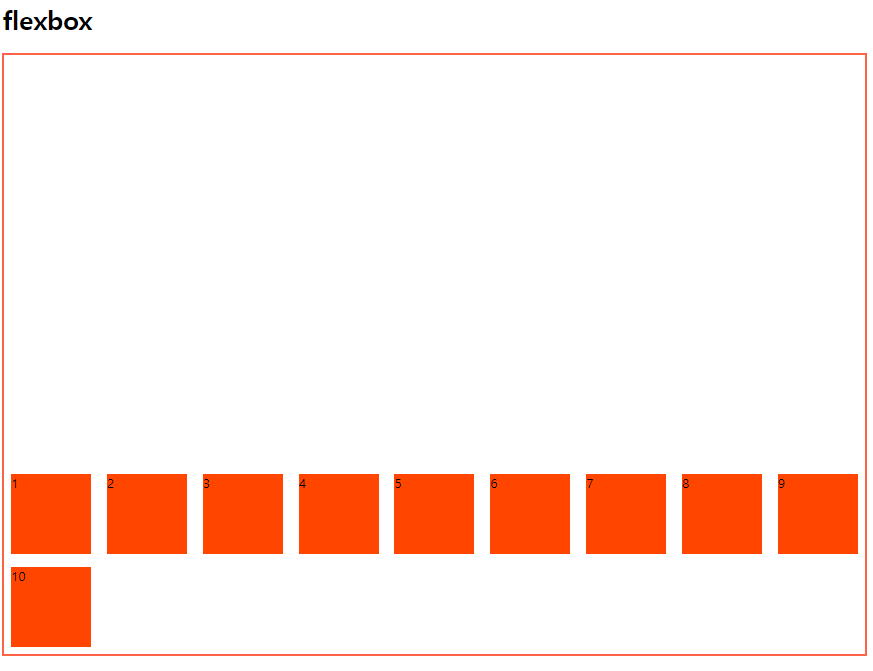
1. column배치, 메인축정렬 center, 줄바꿈wrap, 크로스축정렬 center
2. row배치, 메인축정렬 space-between , 줄바꿈 wrap, 크로스축정렬 center
3. row배치, 메인축정렬 space-between, 줄바꿈 wrap, 크로스축정렬 flex-end
셋 다 flex.
줄바꿈여부를 nowrap으로 할 경우 지정된 크기(100,100픽셀)이 아닌 칸에 맞춰진 크기로 나오게 된다.
메인축과 크로스축 정렬 및 줄바꿈, inline배치 등을 통해서 위와 같이 목록들을 바꿀 수 있다.
transform (바꾸기)
- translate (x축 offset, y축 offset) : 바뀔 위치 지정
- scale (x축 비율, y축 비율)
- rotate (양수는 시계방향, 음수는 반시계방향. 단위 deg(도) )
<h1>transform</h1>
<div class="img-wrapper">
<img src="../sample/image/flower1.PNG" alt="" class="translate">
</div>
<div class="img-wrapper">
<img src="../sample/image/flower2.PNG" alt="" class="scale">
</div>
<div class="img-wrapper">
<img src="../sample/image/flower3.PNG" alt="" class="rotate">
</div>
body{
text-align: center;
}
.img-wrapper{
margin: 100px 0;
}
img{
width: 300px;
}
.translate:hover{
transform: translate(100px,100px);/*x축 offset, y축 offset*/
}
.scale:hover{
transform: scale(1.5,1.5);/*x축비율, y축비율*/
}
.rotate:hover{
transform: rotate(-315deg);/*양수 시계방향, 음수 반시계방향*/
}
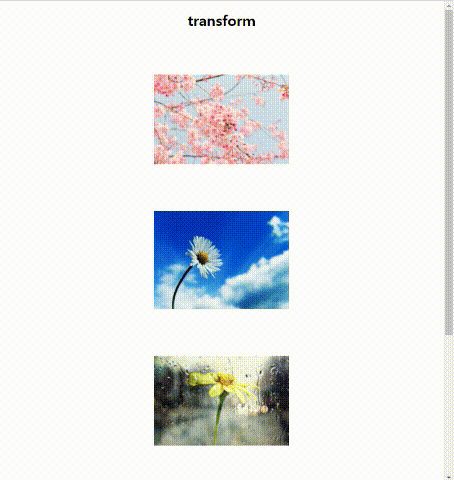
다음과같이 hover하면 위의 것들이 적용된다.
하지만 rotate(-315deg)와 rotate(45deg)가 똑같이 나오게된다. (회전 방향을 알 수 없는것)
이때 필요한 것이 transition이다.
transition (움직임있게 바꾸기)
transition : 하나의 요소가 상태가 변경될 때 변경하는 과정을 노출시켜 동영상 같은 효과를 연출한다.
- transition-duration : (단위 s) 소요시간
- transition-property : all ( 기본값 ) . all이아닌 B상태에서 지정된 요소중 하나로 선언하면, 그것만 바뀐다.
- transition-delay : (단위 s) 지연시간
- transition-timing-function : ease(기본값. 완만하게) | ease-in | ease-out | linear (똑같이)
transition : property , duration, timing-function , delay 순으로 모아쓰기 가능!
overflow : hidden : 요소 바깥 감추기
<div class="box"></div>
<hr>
<!--div.tile>h1+h2.transition+p.transition>lorem-->
<div class="wrapper">
<div class="tile">
<h1>Hello World</h1>
<h2 class="transition">Hello World부터 Lotte World까지...</h2>
<p class="transition">Lorem ipsum dolor sit, amet consectetur adipisicing elit. Sapiente, animi non. Id ex non quibusdam facilis in, rerum repellat, libero eveniet esse, obcaecati a aut dolor corrupti reprehenderit perferendis necessitatibus.</p>
</div>
<div class="tile">
<h1>Hello World</h1>
<h2 class="transition">Hello World부터 Lotte World까지...</h2>
<p class="transition">Lorem ipsum dolor sit, amet consectetur adipisicing elit. Sapiente, animi non. Id ex non quibusdam facilis in, rerum repellat, libero eveniet esse, obcaecati a aut dolor corrupti reprehenderit perferendis necessitatibus.</p>
</div>
<div class="tile">
<h1>Hello World</h1>
<h2 class="transition">Hello World부터 Lotte World까지...</h2>
<p class="transition">Lorem ipsum dolor sit, amet consectetur adipisicing elit. Sapiente, animi non. Id ex non quibusdam facilis in, rerum repellat, libero eveniet esse, obcaecati a aut dolor corrupti reprehenderit perferendis necessitatibus.</p>
</div>
<div class="tile">
<h1>Hello World</h1>
<h2 class="transition">Hello World부터 Lotte World까지...</h2>
<p class="transition">Lorem ipsum dolor sit, amet consectetur adipisicing elit. Sapiente, animi non. Id ex non quibusdam facilis in, rerum repellat, libero eveniet esse, obcaecati a aut dolor corrupti reprehenderit perferendis necessitatibus.</p>
</div>
</div>
.box{
width:100px;
height: 100px;
background-color: tomato;
border: 2px solid #000;
transition-duration: 2s;/*소요시간*/
transition-property: transform;/*all(기본값) , 지정하면 그것만 바뀜 */
transition-delay: .3s;
transition-timing-function: ease;/*ease(기본값.완만하다) | ease-in | ease-out | linear*/
/*property , duration , timing-function , delay */
/*transition: transform 2s linear .3s; 모아쓰기 가능*/
}
body:hover .box{
transform: translate(500px,0);
background-color: yellow;
}
.wrapper{
display:flex;
flex-wrap: wrap;/*카드요소 배치 가로로*/
}
.tile{
width: 380px;
height: 380px;
margin: 10px;
padding: 30px;
background-color: #99aeff;
overflow: hidden;/*부모 요소 밖 감추기*/
box-shadow: 0 35px 77px -17px rgba(0,0,0,.4);
color:white;
}
.tile h1{
text-shadow: 2px 2px 10px rgba(0,0,0, .3);
}
.tile h2{
font-style:italic;
transform: translateX(380px);
}
.tile p{
transform:translateX(-380px);
transition-delay: 0.3s;
}
.transition{
transition-duration: .6s;
opacity: 0.3;
}
.tile:hover{
box-shadow: 0 35px 77px -17px rgba(0,0,0, .8);
}
.tile:hover .transition{
transform: translateX(0);
opacity: 1;
}
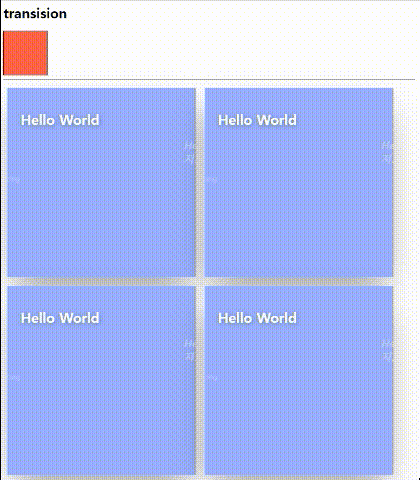
transition-property에서 transform(움직임) 만 지정해줬기때문에 움직이는것은 완만하게 이동하고, 색상은 hover시 바로 바뀌는 것을 알 수 있다.
속도는 2배속을 해서 1초만에 움직이는것으로 보이지만 실제로 2초가 걸린다.
아래 카드의 경우, overflow : hidden으로 글씨가 박스 바깥으로 나간 것은 보이지 않게 설정되었다.
hover시 상자 그림자가 진해지는 것을 확인할 수 있다.
hover시 글자가 양쪽에서 가운데로 이동해오는 것을 확인할 수 있다.
<div class="img-wrapper">
<img src="../sample/image/flower1.PNG" alt="">
</div>
<div class="img-wrapper">
<img src="../sample/image/flower2.PNG" alt="">
</div>
<div class="img-wrapper">
<img src="../sample/image/flower3.PNG" alt="">
</div>
<div class="img-wrapper">
<img src="../sample/image/flower4.PNG" alt="">
</div>
.container {
display: flex;
flex-wrap: wrap;
}
.img-wrapper{
width: 300px;
height: 200px;
border: 1px solid lightgray;
overflow: hidden;
margin: 3px;
}
.img-wrapper img{
width: 100%;
transition-duration: .3s;
}
.img-wrapper:hover img{
transform: scale(1.1);
}
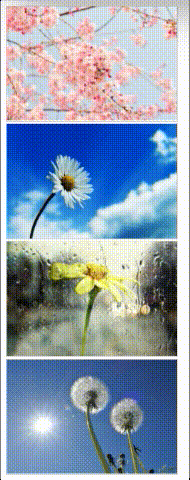
이 역시 overflow : hidden으로 사진이 1.1배 확대되지만, 박스 바깥은 뜨지 않는 것을 확인할 수 있었다.
하지만 transition은 상태 두가지를 변화시킬 수 밖에 없는데, 여러개의 상태를 이용하여 애니메이션처럼 바뀌게도 할 수 있다!
animation (상태 A,B반복이 아닌 여러가지 동작 이어서 하기)
- @keyframes 프레임명 : 여러개의 상태를 표현
- from ~ to : A상태에서 B 로 (transition이랑 같음)
- 0% 25% 50% 75% 100% : %로 여러 상태를 연결해 표현이 가능
- animation : 프레임명 keyframes 의 속성명
- animation-dufation : (단위s) 변경에 걸리는 시간
- animation-direction : 애니메이션 방향
- animation-delay : (단위 s)딜레이시간
- animation-iteration-count : infinite(기본값) 애니메이션 지속여부
- animation-tiiming-function : ease(기본값) | linear 변경속도옵션
- animation-fill-mode : forwards | backwards 애니메이션 시작 전 후 머무를 위치
animation : name duration timing-function delay iteration-count direction fill-mode 순으로 한번에 작성가능
<div class="box"></div>
<img src="../sample/image/hyunta.jpg" alt="" id="mydog">
#mydog{
width: 100px;
border-radius: 50%;
margin: 50px;
}
body:hover #mydog{
animation: myframes 2s infinite alternate-reverse;
}
.box{
width:100px;
height: 100px;
background-color: hotpink;
border:1px solid #000;
margin: 50px;
}
body:hover .box{
animation: myframes; /*@keyframes 속성명*/
animation-duration: 3s;
/* animation-iteration-count: infinite; */
animation-direction: normal;
animation-delay: .5s;
animation-timing-function: linear;/*ease(기본값)*/
animation-fill-mode: forwards ;/* 애니매이션 시작전후 머무르게 될 위치 : forwoards (시작부분) , backwards (끝나는부분에서끝)*/
/*name duration timing-function delay iteration-count direction fill-mode */
animation: myframes 3s ease .5s infinite forwards;
}
body{
height: 100vh;
}
@keyframes myframes {
25%{
transform: translate(200px,0);
}
50%{
transform: translate(200px,200px);
}
75%{
transform: translate(400px,200px);
}
100%{
transform: translate(400px,400px);
}
}
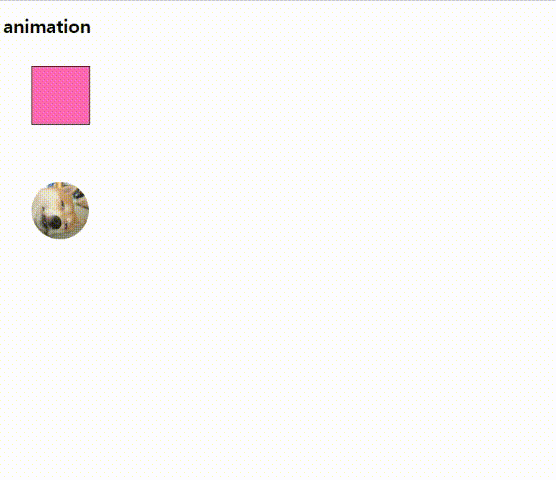
위치를 계단모양으로 바꿔가면서 이동하는 것을 확인할 수 있다.
강아지의 경우 alternate-reverse를 통해 거꾸로 실행됨을 확인할 수 있다.
layout
html5 이전 레이아웃
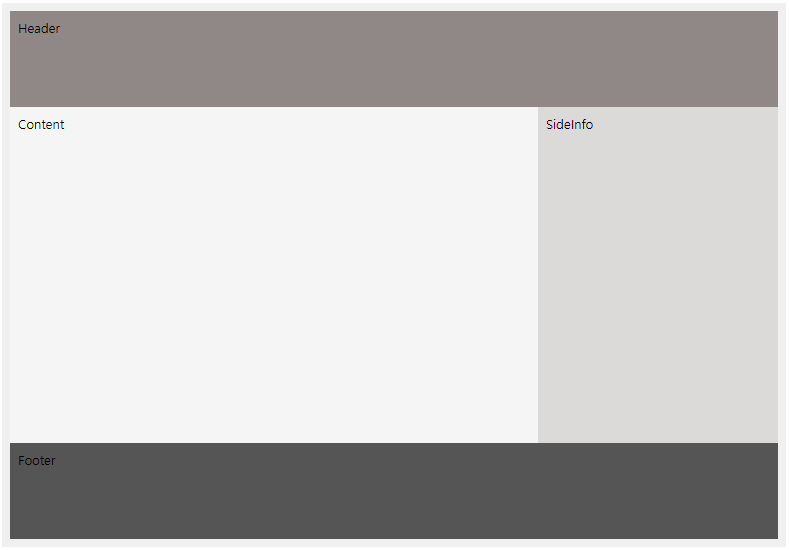
html5 레이아웃
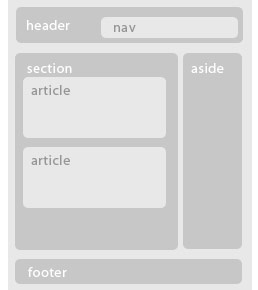
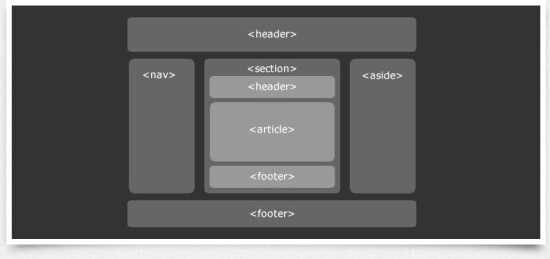
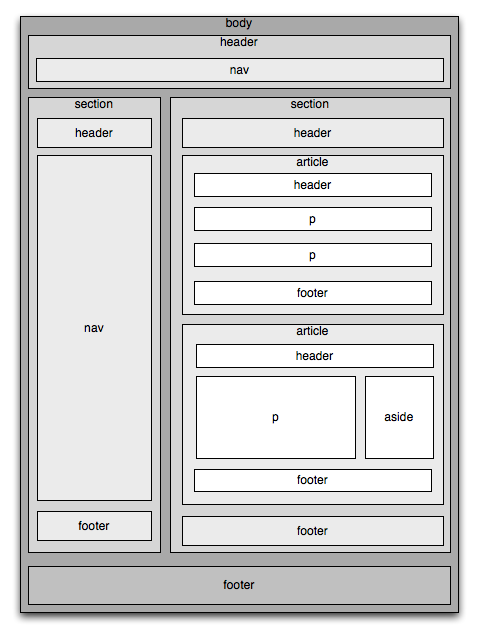
- semantic tag
- 태그의 내용과 일치하는 태그를 사용할 것.
- screen reader에서 태그를 통해 본문의 내용을 조회 / 검색. 웹 접근성을 향상시킴
<header></header>
- 사이트전체의 헤더영역, article태그의 헤더영역
<main></main>
- 본문영역으로 한 페이지에서 단 한번만 사용해야 한다.
<article></article>
- 웹페이지의 실제내용. 포스팅, 신문/잡지등의 기사 등.
- 문서내에 여러개의 article이 존재할 수 있다.
- 내부적으로 header, section, footer등으로 구성될 수 있다.
<section></section>
- 연관된 컨텐츠를 그룹핑.
- 내부에 여러 article, p태그등을 가질 수 있다.
- div는 css적인 효과를 위해 그룹핑하는 반면, section은 내용위주로 그룹핑한다.
<nav></nav>
- 네비게이션용 태그.
- 위치가 가장 자유롭다. header안, aside안, footer안, nav단독으로 사용가능
<aside></aside>
- 부가정보를 표현하는 영역. 광고, 날씨, navigation정보...
<footer></footer>
- 사이트하단영역 또는 article태그의 footer영역. 사이트맵, 저작권정보, 연락처 등.